Background.
I was asked to put together a 2 day class on the internet of things at PNCA so I suggested that we try out the Adafruit huzzah. while I was looking for it I came across the Sparkfun Esp8266 “thing” and the Esp8266 dev board. I used the Sparkfun Esp8266 dev board to implement a light socket that queried the internet for its State.
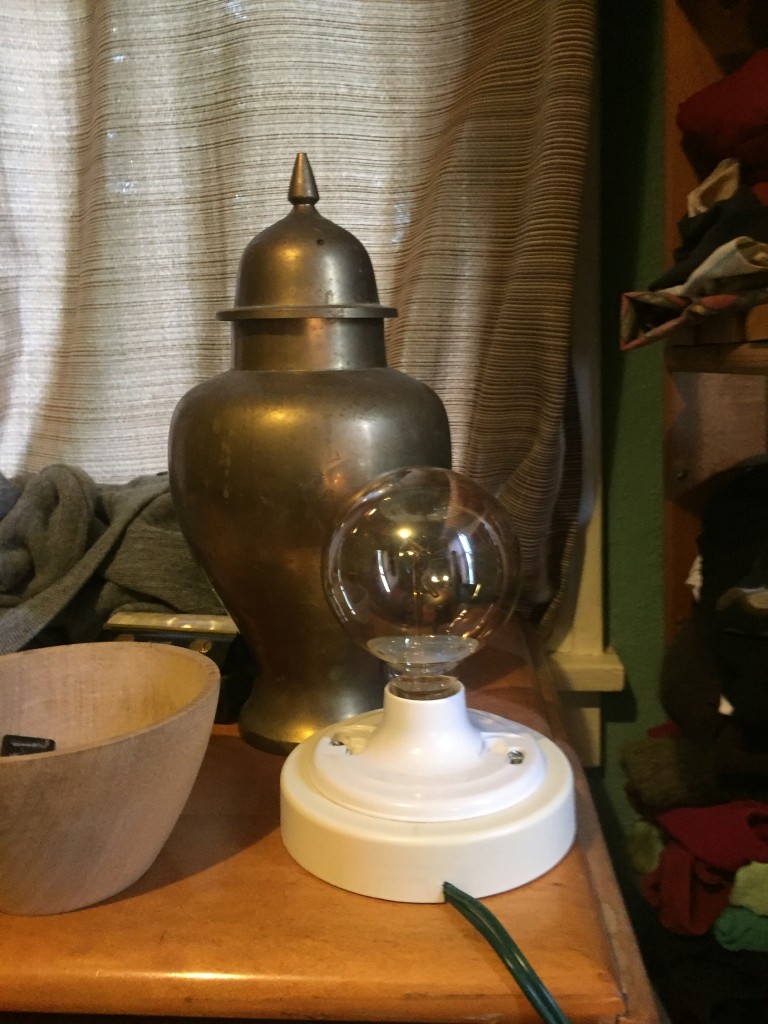
Connections
The most important thing you can do with your Huzzah is to make sure you have at least 250 ma of power available to the wifi part of the module. There is nothing more frustrating than having everything worked as advertised until you turn the radio on. The Sparkfun boards have usb power which is good enough. The Huzzah has an on board regulator for both external battery and can be powered by USB’s 5v and it detects an d uses whichever is greater.
After working through some code on the Huzzah with the students, I went to the Hardware store and purchased and externally mounted light socket, I had brought with me a solid state relay from my bench pile. For all practical purposes an SSR can be thought of as an LED. In fact the SSR that I chose did not have a current reducing circuit and I let the smoke out of the first one. To power the board I purchased a mini usb charger which without the external casing fit fine in the enclosure.
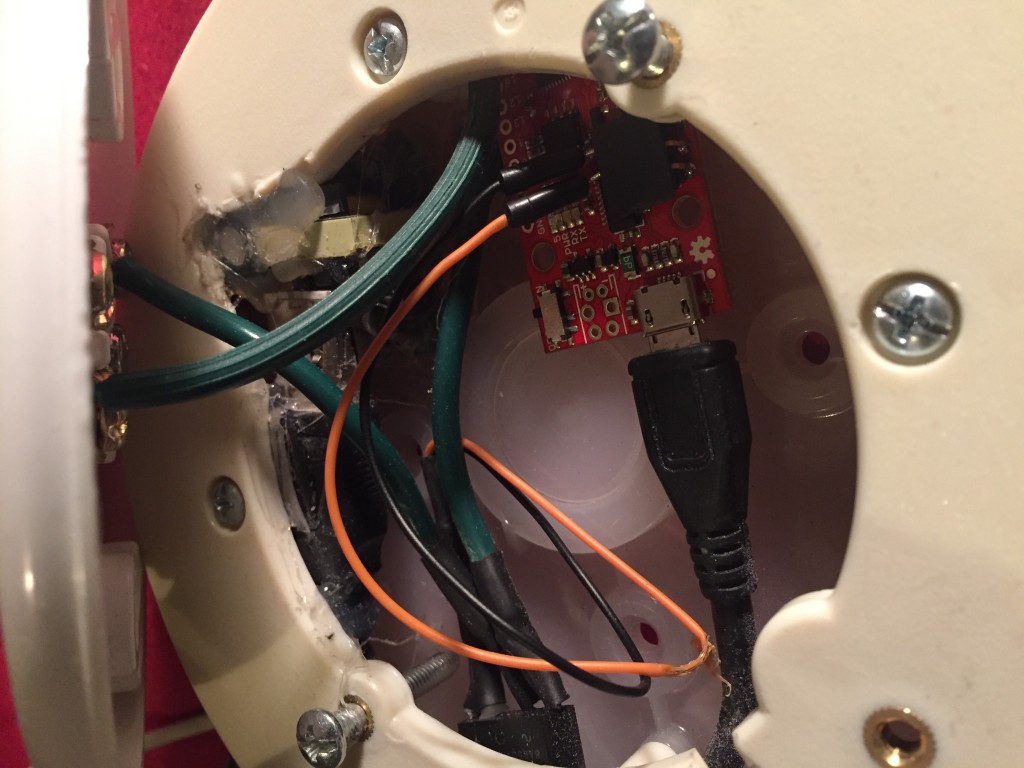
The Server from ByteMe 2013
The web server for the afru show is still presenting the state of Stephen Wrights Light Switch from the AFRU Gallery’s annual Byte Me show in 2013. The code to present the state is a small pile of python. The most important part is the 3 non standard ways to tell your browser to stop caching the data since no-one seems to follow the established standards (..that means you safari..)
...$ cat index.wsgi import webapp2 import sqlite3 class MainPage(webapp2.RequestHandler): def get(self): con=sqlite3.connect('/home/newcourse/suspectdevices/www/art2013/swls/swls.db') cur=con.cursor() cur.execute("select state from switch") swstate=cur.fetchone()[0] self.response.headers['Content-Type'] = 'text/plain' self.response.headers['Cache-Control'] = 'no-cache, no-store, must-revalidate' self.response.headers['Pragma'] = 'no-cache' self.response.headers['Expires'] = '0' self.response.out.write(swstate) application = webapp2.WSGIApplication([('/art2013/swls/index.wsgi', MainPage)], debug=True)
Creating the Light Socket using the esp8266 dev board
The arduino code for creating the light switch is remarkably simple. After setting up the wifi connection the device repeatedly does does an http get which returns the header information and a body containing either ON or OFF. Since the standard headers do not contain the word ON we look for that using the arduino String class’ indexOf method.
/*----------------------FrauleinMartinaFranksLightSocket.ino This is a hack of the provided WifiClient example. This is free software (BSD License) (C) 2015 Donald Delmar Davis , SuspectDevices. ----------------------------------------------------------*/ #include <ESP8266WiFi.h> /* Declarations and constants */ #define ESP8266_DEV_BOARD 1 #define SWITCH_OFF 1 #define SWITCH_ON 0 #define LED_OFF 0 #define LED_ON 1 #define RELAY_OFF 0 #define RELAY_ON 1 const char* ssid = "MYSSID"; const char* password = "myWifiPassword"; const char* host = "www.suspectdevices.com"; const int httpPort = 80; const char* offstate = "OFF"; const char* onstate = "ON"; #ifdef ESP8266_DEV_BOARD // esp8266 dev board 0,4,5(LED) and 14 are available as General Purpose pins // on board led is at pin 5 // avoid pin 15 messes with downloading const int switchPin = 4; const int ledPin = 5; const int relayPin = 2; #elseif defined(ADAFRUIT_HUZZAH) const int switchPin = 4; const int ledPin = 13; const int relayPin = 2; #else const int switchPin = 4; const int ledPin = 13; const int relayPin = 2; #endif /* global variables */ bool oldSwitchValue = SWITCH_OFF; /*-----------------------------------------------------------setup() */ void setup() { bool flipFlop = SWITCH_OFF; Serial.begin(115200); pinMode(switchPin, INPUT_PULLUP); pinMode(ledPin, OUTPUT); digitalWrite(ledPin, LED_ON); pinMode(relayPin, OUTPUT); digitalWrite(relayPin, RELAY_ON); delay(10); // We start by connecting to a WiFi network Serial.println(); Serial.println(); Serial.print("Connecting to "); Serial.println(ssid); WiFi.begin(ssid, password); while (WiFi.status() != WL_CONNECTED) { flipFlop = !flipFlop; digitalWrite(ledPin, flipFlop); delay(500); Serial.print("."); } digitalWrite(ledPin, LED_OFF); Serial.println(""); Serial.println("WiFi connected"); Serial.println("IP address: "); Serial.println(WiFi.localIP()); } /*---------------------------------------------------loop() */ void loop() { delay(1000); Serial.print("connecting to "); Serial.println(host); // Use WiFiClient class to create TCP connections WiFiClient client; if (!client.connect(host, httpPort)) { Serial.println("connection failed"); return; } // We now create a URI for the request String url = "/art2013/swls/"; Serial.print("Requesting URL: "); Serial.println(url); // This will send the request to the server client.print(String("GET ") + url + " HTTP/1.1\r\n" + "Host: " + host + "\r\n" + "Connection: close\r\n\r\n"); for (int t = 5; t > 0 && !client.available(); t--) delay(100); // Read all the lines of the reply from server and print them to Serial while (client.available()) { String line = client.readStringUntil('\r'); if (line.lastIndexOf("ON") >= 0) { Serial.println("FOUND AN ON!!!"); oldSwitchValue = SWITCH_ON; } else { oldSwitchValue = SWITCH_OFF; } Serial.print(line); } digitalWrite(ledPin, oldSwitchValue ? LED_ON : LED_OFF); digitalWrite(relayPin, oldSwitchValue ? RELAY_ON : RELAY_OFF); Serial.println(); Serial.println("closing connection"); }

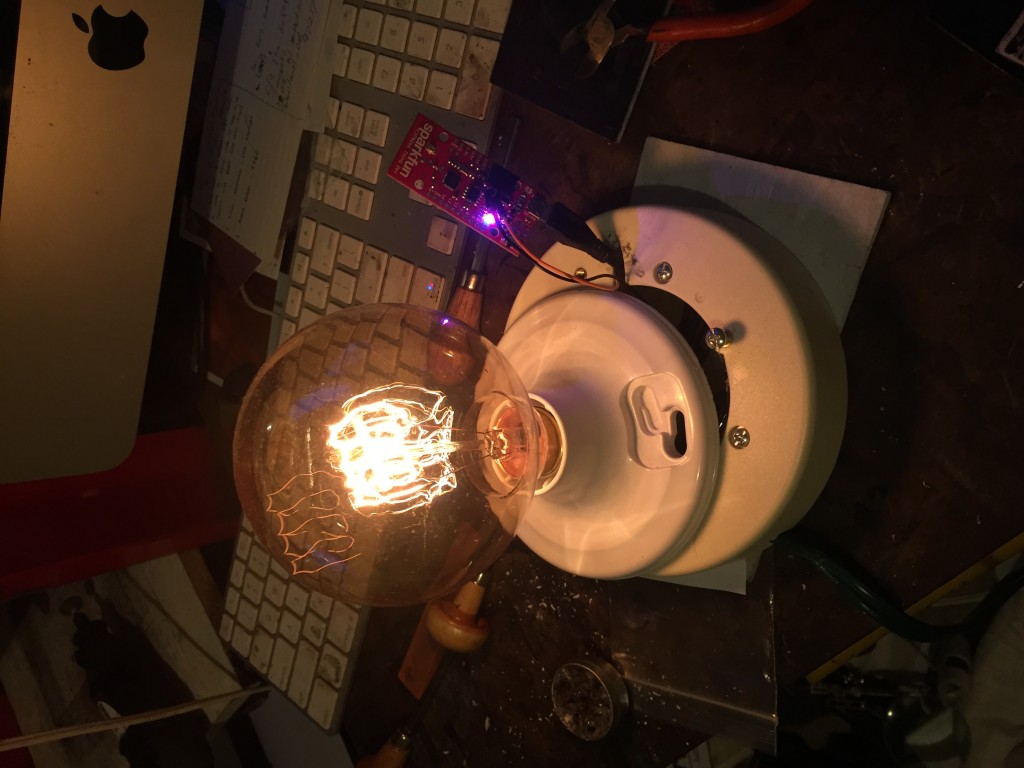